Python and Java are two of the world’s most widely used programming languages. Each has its strengths, weaknesses, and specific areas where it excels. Choosing between Python and Java can be difficult, as both languages offer unique benefits depending on the context of their use.
This article aims to comprehensively compare Python and Java, examining their syntax, performance, use cases, community support, and more. By understanding the key differences and similarities, you can decide which language is best suited for your project or career goals.
Syntax and Ease of Use
Python
Python is renowned for its simplicity and readability. Its syntax is clean and straightforward, which makes it an excellent choice for beginners. The language emphasizes code readability and uses indentation to define code blocks, eliminating the need for curly braces or semicolons.
# Python Example
def greet(name):
print(f"Hello, {name}!")
greet("World")
PythonPython’s dynamic typing and lack of explicit declarations make it a highly flexible language. However, this can also lead to runtime errors if not carefully managed.
Java
On the other hand, Java is a statically typed language, meaning that all variables must be declared before they can be used. This makes Java code more verbose but also helps catch errors at compile time rather than at runtime.
// Java Example
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
JavaJava’s syntax is more complex than Python’s, but it provides a robust structure beneficial for large-scale projects. Using explicit type declarations can make the code easier to understand and maintain.
Performance
Python
Python is an interpreted language, generally slower than compiled languages like Java. However, Python’s performance is often sufficient for many applications, mainly when focused on development speed and ease of use.
Python’s Global Interpreter Lock (GIL) can be a bottleneck for multi-threaded applications, as it allows only one thread to execute at a time. This limitation can be mitigated by multiprocessing or integrating Python with other languages for performance-critical components.
Java
Java is a compiled language on the Java Virtual Machine (JVM). This allows Java to perform well across various platforms. Java’s Just-In-Time (JIT) compiler optimizes code execution at runtime, enhancing performance significantly.
Java is well-suited for multi-threaded applications due to its built-in concurrency features and robust memory management. This makes Java a preferred choice for high-performance applications, such as large-scale enterprise systems and real-time applications.
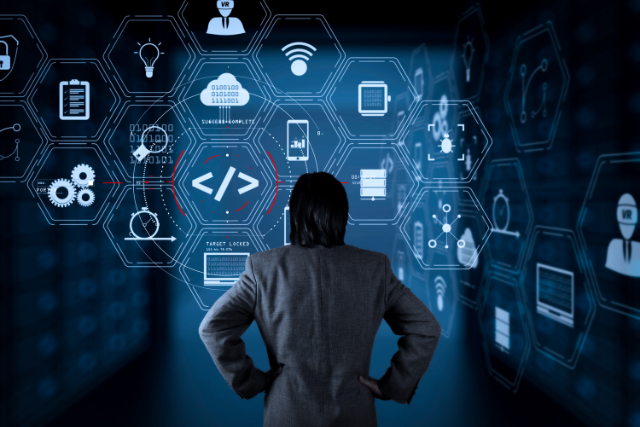
Use Cases
Python
Python’s versatility and ease of use have made it a popular choice in various industries:
Data Science and Machine Learning
Python dominates the data science and machine learning fields due to its powerful libraries like NumPy, pandas, and TensorFlow. Its ability to handle large datasets, perform complex calculations, and build predictive models makes it indispensable for data scientists and machine learning engineers.
Web Development
Frameworks like Django and Flask have made Python a popular choice for web development. Django, in particular, provides a high-level framework that encourages rapid development and clean, pragmatic design, while Flask offers simplicity and flexibility for smaller projects.
Automation and Scripting
Python’s simplicity and readability make it ideal for writing scripts to automate repetitive tasks. It is widely used for automating system administration, data processing, and testing workflows.
Education
Python’s easy-to-learn syntax and extensive community support make it a popular language for teaching programming in schools and universities. Platforms like Codecademy and Coursera offer comprehensive Python courses, making it accessible to learners of all levels.
Java
Java’s robustness, performance, and scalability have cemented its place in various industries:
Enterprise Applications
Java is widely used in enterprise environments due to its stability, scalability, and security features. Frameworks like Spring and Hibernate facilitate the development of complex enterprise applications, making Java a go-to choice for large organizations.
Android Development
Java is the primary language for Android app development, supported by the Android SDK. Its extensive libraries and tools enable developers to build robust, feature-rich mobile applications.
Financial Services
Java’s performance and reliability make it ideal for financial and banking applications. It is used for developing trading platforms, transaction processing systems, and risk management solutions.
Web Development
Java’s web development frameworks, such as Spring MVC and Apache Struts, provide robust solutions for building scalable web applications. Java’s strong typing and compile-time error checking contribute to developing secure and maintainable code.
Community and Support
Python
Python has a vast and active community that contributes to a wealth of libraries, frameworks, and tools. The Python Package Index (PyPI) hosts thousands of packages that can be easily integrated into projects, accelerating development.
The community support is reflected in the numerous tutorials, forums, and online courses available for learning Python. The Python Software Foundation also plays a significant role in promoting and supporting the language.
Java
Java also boasts a large and mature community with extensive resources available for learning and development. The Java Community Process (JCP) allows developers to participate in the evolution of the language, ensuring it meets the needs of its users.
Java’s long-standing presence in the industry means many libraries, frameworks, and tools are available. Resources such as Stack Overflow, GitHub, and numerous online courses provide ample support for Java developers.
Development Speed
Python
Python is often praised for its rapid development cycle. The language’s simplicity and ease of use allow developers to write and test code quickly. This makes Python a preferred choice for prototyping, startups, and projects where time-to-market is critical.
The availability of extensive libraries and frameworks further accelerates development, enabling developers to focus on implementing features rather than writing boilerplate code.
Java
Java’s development cycle can be longer compared to Python due to its verbosity and the need for explicit type declarations. However, Java’s robust tooling and integrated development environments (IDEs) like IntelliJ IDEA, Eclipse, and NetBeans provide powerful productivity features.
Java’s strong typing and compile-time error checking can reduce runtime errors, resulting in more stable and maintainable code, saving time in the long run.
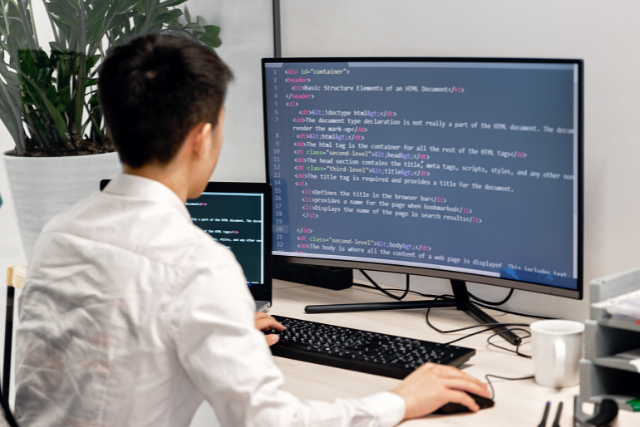
Ecosystem and Libraries
Python
Python’s ecosystem has libraries and frameworks catering to various domains. Some notable examples include:
- Django and Flask: Popular web frameworks that simplify web application development.
- NumPy and pandas: Essential libraries for data manipulation and analysis.
- TensorFlow and PyTorch: Leading frameworks for machine learning and artificial intelligence.
- Beautiful Soup and Scrapy: Tools for web scraping and data extraction.
Python’s versatility and the availability of these libraries make it a powerful tool for a wide range of applications.
Java
Java’s ecosystem is also extensive, with a strong focus on enterprise and large-scale applications. Some essential libraries and frameworks include:
- Spring: A comprehensive framework for building enterprise applications.
- Hibernate: An object-relational mapping (ORM) framework for database interactions.
- Apache Maven and Gradle: Build automation tools that streamline project management and dependency resolution.
- JUnit: A widely used testing framework for Java applications.
Java’s mature ecosystem supports the development of complex and robust applications across various domains.
Learning Curve
Python
Python is often recommended as the first programming language for beginners due to its simplicity and readability. The language’s straightforward syntax allows new programmers to focus on learning programming concepts without getting bogged down by complex syntax rules.
The abundance of learning resources, including online tutorials, courses, and books, makes it easy to start with Python. Community-driven platforms like Stack Overflow and GitHub provide valuable support for learners.
Java
Java’s learning curve can be steeper compared to Python, especially for beginners. The language’s verbosity and the need to understand object-oriented programming (OOP) concepts can be challenging for new programmers.
However, Java’s structured approach and strong typing can provide a solid foundation for learning programming. The extensive documentation and resources available for Java allow learners to overcome the initial hurdles and become proficient.
Python and Java Development Tools
Python Development Tools
1. PyCharm
PyCharm is an integrated development environment (IDE) specifically for Python development, developed by JetBrains. It offers a wide range of features to facilitate efficient coding, debugging, and testing.
Key Features:
- Code Completion and Navigation: PyCharm provides intelligent code completion, on-the-fly error checking, and quick fixes. It also offers powerful navigation features to jump to definitions, usages, and classes.
- Debugging and Testing: The IDE includes a powerful debugger and supports unit testing with frameworks like pytest and unittest.
- Integration with Tools: PyCharm integrates seamlessly with tools like Docker, Vagrant, and databases, providing a comprehensive development environment.
- Refactoring: It offers a wide range of refactoring tools, making it easy to improve and maintain code quality.
2. Jupyter Notebook
Jupyter Notebook is an open-source web application that allows you to create and share documents containing live code, equations, visualizations, and narrative text.
Key Features:
- Interactive Computing: Jupyter Notebook supports interactive data visualization and manipulation, making it a popular tool in data science and machine learning.
- Multi-language Support: While primarily used for Python, Jupyter supports other programming languages like R and Julia through its extensible kernel architecture.
- Visualization Libraries: Integration with libraries like Matplotlib, Plotly, and Seaborn allows for rich, interactive visualizations.
- Collaboration: Notebooks can be shared easily, making them ideal for collaboration and educational purposes.
3. Anaconda
Anaconda is a distribution of Python and R for scientific computing and data science. It simplifies package management and deployment.
Key Features:
- Package Management: Anaconda includes Conda, a package manager that simplifies the installation and management of libraries and dependencies.
- Pre-installed Libraries: It comes with hundreds of pre-installed libraries for data science, including NumPy, pandas, and scikit-learn.
- Integrated Development: Anaconda includes Jupyter Notebook and other tools like Spyder, providing a comprehensive environment for data science.
- Cross-platform Support: Available for Windows, macOS, and Linux, Anaconda ensures a consistent development environment across different platforms.
Java Development Tools
1. IntelliJ IDEA
IntelliJ IDEA is an integrated development environment developed by JetBrains, designed for JVM languages, including Java. It is known for its powerful features and intelligent code assistance.
Key Features:
- Smart Code Completion: IntelliJ IDEA provides context-aware code completion, significantly speeding up coding.
- Powerful Refactoring Tools: The IDE offers advanced refactoring tools, helping developers improve and maintain code quality.
- Integrated Build Tools: It supports build tools like Maven, Gradle, and Ant, streamlining project management and dependency handling.
- Robust Debugging: IntelliJ IDEA includes a powerful debugger with features like breakpoints, watches, and variable evaluation.
- Plugin Ecosystem: A vast plugin ecosystem allows for extensive customization and enhancement of the development environment.
2. Eclipse
Eclipse is a popular open-source IDE primarily used for Java development. It provides a versatile and customizable development environment.
Key Features:
- Extensible Plugin System: Eclipse’s plugin architecture allows developers to add functionalities tailored to their specific needs.
- Robust Debugging and Profiling: The IDE includes powerful debugging and profiling tools to identify and resolve performance issues.
- Integrated Development: Eclipse supports multiple languages through plugins, making it a versatile tool for polyglot developers.
- Version Control Integration: It integrates with version control systems like Git and SVN, facilitating collaborative development.
3. NetBeans
NetBeans is an open-source IDE for Java development, providing comprehensive tools for developing desktop, web, and mobile applications.
Key Features:
- Project Management: NetBeans offers robust project management features, including support for multiple project types and configurations.
- Smart Code Editing: The IDE provides code completion, templates, and refactoring tools to enhance productivity.
- Cross-platform Support: NetBeans is available for Windows, macOS, and Linux, ensuring a consistent development environment.
- Java EE Support: It includes tools and libraries for developing enterprise Java applications, making it ideal for large-scale projects.
Conclusion
Python and Java are powerful programming languages with unique strengths and weaknesses. The choice between them depends on the specific requirements of your project, your familiarity with the languages, and your long-term goals.
Python’s simplicity and versatility make it an excellent choice for rapid development, data science, and scripting. Its extensive library support and active community enhance its appeal for various applications.
Java’s robustness, performance, and scalability make it ideal for large-scale enterprise applications, Android development, and financial services. Its strong typing and compile-time error checking contribute to more stable and maintainable code, making it a reliable choice for complex projects.
Ultimately, both languages have proven their worth in the programming world. By understanding the key differences and similarities between Python and Java, you can make an informed decision that aligns with your project’s needs and career aspirations.
FAQs
1. Which language is better for web development: Python or Java?
Python and Java are suitable for web development but excel in different areas. Python’s Django and Flask frameworks are famous for building web applications quickly and efficiently. Java’s Spring framework is widely used for enterprise-level web applications that require robustness and scalability.
2. Is Python faster than Java?
Generally, Java is faster than Python because Java is a compiled language, while Python is interpreted. Java’s Just-In-Time (JIT) compiler optimizes code execution at runtime, enhancing performance. However, Python’s speed is often sufficient for many applications, and its development speed can offset performance limitations.
3. Which language is easier to learn: Python or Java?
Python is generally considered easier to learn due to its simple and readable syntax. It is often recommended as the first programming language for beginners. With its verbosity and the need to understand object-oriented programming concepts, Java has a steeper learning curve but provides a solid foundation for learning programming.
4. Can Python be used for mobile app development?
While Python is not traditionally used for mobile app development, frameworks like Kivy and BeeWare allow developers to build mobile applications in Python. However, Java remains the primary language for Android app development, supported by the Android SDK.
5. Which language has better job prospects: Python or Java?
Python and Java offer excellent job prospects but cater to different industries. Python is highly demanded for data science, machine learning, web development, and automation. Java is widely used in enterprise applications, Android development, and financial services. Your choice should depend on your career goals and the industry you want to work in.